What you will do:
Write code to control the buzzer and create a simple tune.
What you will learn:
How to set different notes and the length they play for.
How do you write musical notes in code?
In sheet music, each note is on a line or space which shows which note it is, and is a different shape to show how long it is. When we are giving instructions to the buzzer, we need to give it both of these pieces of information as numbers.
Here's a bit of sheet music. It's four beats of middle C, shown on the treble clef.
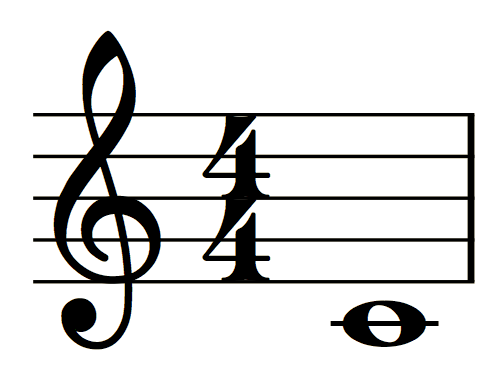
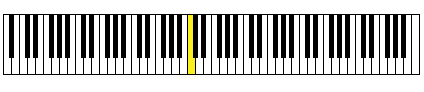
If we wanted to talk to the buzzer we could use a line like:
rainbowhat.buzzer.midi_note(60, 4)
The bit that says "midi_note" says that we are using a system of numbering the notes in order. Middle C is note number 60, and in the example above we've chosen to play it for 4 beats (like in the sheet music).
Writing the code
Open a new project in Mu or Thonny IDE and let's get going by making sure we have the rainbowhat and time modules (in case we want to wait between notes).
import rainbowhat
import time
rainbowhat.buzzer.midi_note(60, 1)
The code will make the buzzer play Middle C for one beat. Let's add a few more notes.
rainbowhat.buzzer.midi_note(64, 1)
rainbowhat.buzzer.midi_note(67, 1)
rainbowhat.buzzer.midi_note(60, 1)
Run the code
Run your code by pressing "play" in Mu or Thonny. Listen to the sounds.
Next Steps
Create a tune of your own.
Change the length of the notes.
Add pauses using time.sleep(1) or different length pauses.