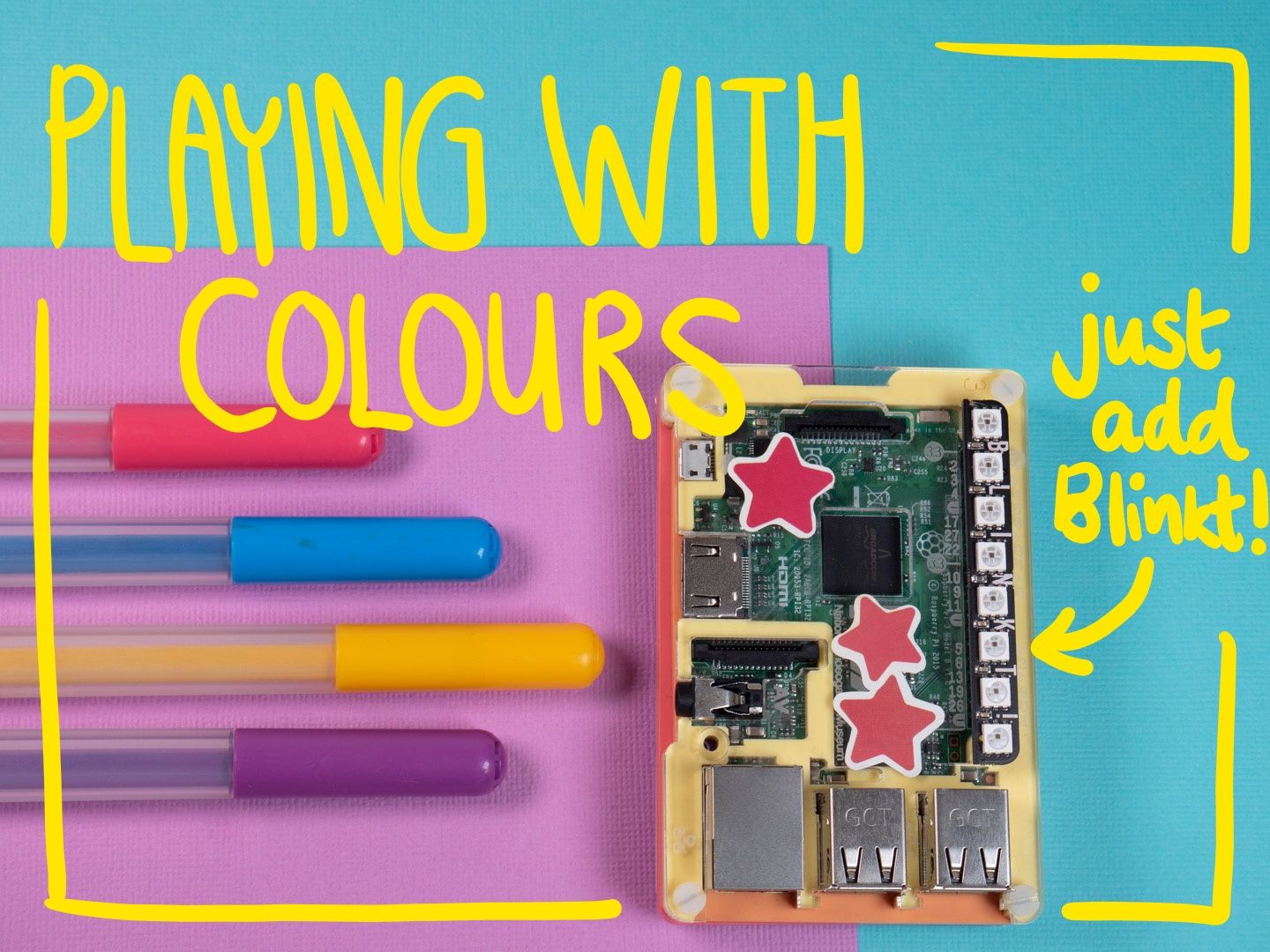
Our Blinkt board is a really quick way to get started with physical computing - instant shiny feedback on your Python coding skills!
When you install the Blinkt library, a number of examples are installed, you'll find them in Pimoroni/blinkt/examples - this activity uses solid_colours.py .
#!/usr/bin/env python
import time
import blinkt
blinkt.set_clear_on_exit()
step = 0
while True:
if step == 0:
blinkt.set_all(128, 0, 0)
if step == 1:
blinkt.set_all(0, 128, 0)
if step == 2:
blinkt.set_all(0, 0, 128)
step += 1
step %= 3
blinkt.show()
time.sleep(0.5)
You'll notice that this code has no comments, so we're going to see if we can figure out what it is doing. The first line tells you that the program is written in Python, a programming language that we can edit using many different editors, but we're going to use Thonny Python IDE, which you can find in the Programming menu on your Raspberry Pi.
Task - Open and Run a Program
Open the program "solid_colours.py" from inside Thonny. You'll need to click on the Pimoroni folder, then on blinkt, and then on examples, before you find it.
Make sure the Blinkt is pushed onto the Raspberry Pi pins the right way up, then use the play button to run the program and see what it does.
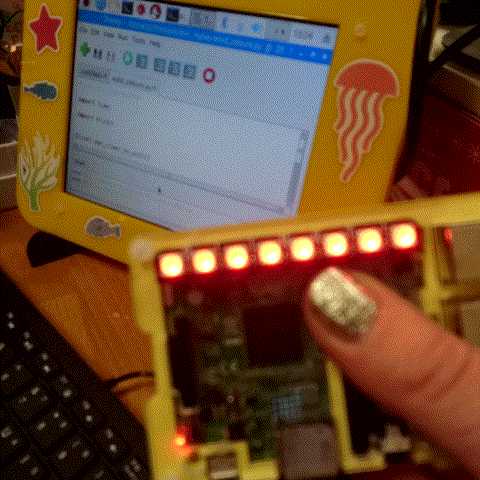
You should see it flashes a pattern of colours. Colours in this program are represented by what we call a tuple (three values in brackets). See if you can find them in this program.
while True:
if step == 0:
blinkt.set_all(128, 0, 0)
if step == 1:
blinkt.set_all(0, 128, 0)
if step == 2:
blinkt.set_all(0, 0, 128)
If you haven't already, press stop on the program to stop the lights flashing.
Task - Alter an Existing Program
Now try changing some of the numbers in the brackets that represent the colours. Press play and run the program again. What has happened?
Task - Find the colour codes for the eight main colour mixes.
You can only use the number 0 or the number 100. Try out different combinations and see if you can find the primary colours of light (red, green and blue), the secondary colours of light (yellow, magenta, and cyan), all of the light colours (white) and no light (black/off).
That's it! You've mixed colours of light!
Now you can use the colours to make patterns, or find out how it steps through the colours in this program.
Download this activity as a pdf worksheet for the classroom here.